#!/usr/bin/env python
# coding: utf-8
# In[1]:
from awesome_panel_extensions.awesome_panel.notebook import Header
Header(folder="examples/reference/frameworks/fast", notebook="FastLiteralInput.ipynb")
# # Fast LiteralInput - Reference Guide
#
# The `FastLiteralInput` widget is based on the [fast-text-field](https://explore.fast.design/components/fast-text-field) web component and extends the built in [Panel LiteralInput](https://panel.holoviz.org/reference/widgets/LiteralInput.html) widget.
#
#
#
# 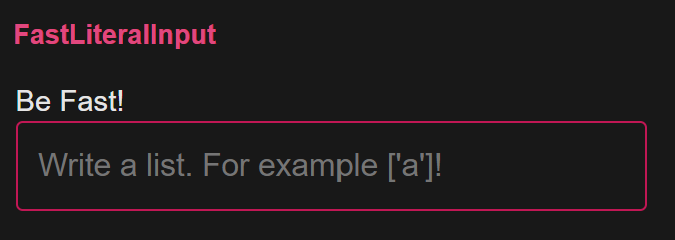 |
#
#
#
#
# #### Parameters:
#
# ##### Core
#
# * **``value``** (str): Parsed value of the indicated type
# * **``type``** (type or tuple(type)): A Python literal type (e.g. list, dict, set, int, float, bool, str)
# * **``serializer (str['ast', 'json])``**: The serialization (and deserialization) method to use. 'ast' uses ast.literal_eval and 'json' uses json.loads and json.dumps.
#
# ###### Display
#
#
# * **``name``** (str): The label of the LiteralInput.
# * **``placeholder``** (string): A placeholder string displayed when no value is entered.
# * **``apperance``** (string): Determines the appearance of the LiteralInput. One of `outline` or `filled`. Defaults to `outline`.
# * **``autofocus``** (bool): The autofocus attribute. Defaults to `False`.
# * **``disabled``** (boolean): Whether or not the LiteralInput is disabled. Defaults to False.
# * **``readonly``** (bool): Whether or not the LiteralInput is read only. Defaults to `False`.
#
#
#
# The `FastLiteralInput` has the same layout and styling parameters as most other widgets. For example `width` and `sizing_mode`.
#
# Please note that you can only use the Fast components inside a custom Panel template that
#
# - Loads the [Fast `javascript` library](https://www.fast.design/docs/components/getting-started#from-cdn).
# - Wraps the content of the `` html tag inside the [fast-design-system-provider](https://www.fast.design/docs/components/getting-started#add-components) tag.
#
# We provide the `FastTemplate` for easy usage.
#
# You can also develop your own custom [Panel template](https://panel.holoviz.org/user_guide/Templates.html) if you need something special. For example combining it with more [fast.design](https://fast.design/) web components and the [Fluent Design System](https://www.microsoft.com/design/fluent/#/) to create **VS Code** and **Microsoft Office** like experiences.
#
# Please note that Fast components will not work in older, legacy browser like Internet Explorer.
#
# ___
#
# Let's start by importing the dependencies
# In[2]:
import param
import panel as pn
from awesome_panel_extensions.frameworks.fast import FastTemplate, FastLiteralInput
pn.config.sizing_mode = "stretch_width"
pn.extension()
# ## Parameters
#
# Let's explore the parameters of the `FastLiteralInput`.
# In[3]:
LiteralInput = FastLiteralInput(name="List Input", sizing_mode="fixed", width=300, appearance="outline", placeholder="Input a list. For example ['a', 'b']", type=list)
app=pn.Row(
LiteralInput
)
template=FastTemplate(main=[app])
template
# In[4]:
LiteralInput_parameters = ["name", "value", "disabled", "placeholder", "appearance", "autofocus", "readonly", "height", "width", "sizing_mode"]
settings_pane = pn.WidgetBox(pn.Param(LiteralInput, parameters=LiteralInput_parameters, show_name=False))
settings_pane
# ## pn.Param
#
# Let's verify that that `FastLiteralInput` can be used as a widget by `pn.Param`.
# In[5]:
import param
import panel as pn
WIDGETS = {
"a_literal": {
"type": LiteralInput, "sizing_mode": "fixed", "width": 400, "placeholder": "Input a dictionary!"
}
}
class ParameterizedApp(param.Parameterized):
a_literal = param.Dict(default=None, label="A Dictionary")
view = param.Parameter()
def __init__(self, **params):
super().__init__(**params)
self.view = pn.Param(self, parameters=["a_literal"], widgets=WIDGETS)
parameterized_app = ParameterizedApp()
paremeterized_template = FastTemplate(main=[parameterized_app.view])
paremeterized_template
# In[6]:
pn.Param(parameterized_app.param.a_literal)
# ## Resources
#
# - [fast.design](https://fast.design/)
# - [fast-text-field](https://explore.fast.design/components/fast-text-field)
#
# ## Known Issues
#
# - The `fast-text-field` web component has additional attributes (`max_length`, `min_length`, `pattern`, `size`, `spellcheck`, `required`) that does not seem to work. If you think they are important please upvote [Fast Github Issue 3852](https://github.com/microsoft/fast/issues/3852).
# - It's not possible to specify the `type` of literal in a `pn.Parameterized` class. See [Panel Github 1574](https://github.com/holoviz/panel/issues/1574)
#
#
#
#  |
#
#
#